Git Good
Linus Torvalds’ Magical Time Machine
1 Keeping track of files is hard
1.1 We’ve all seen it before
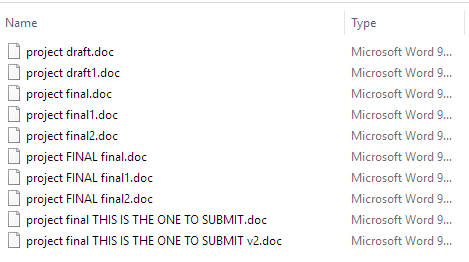
1.2 We’ve all seen it before (2)
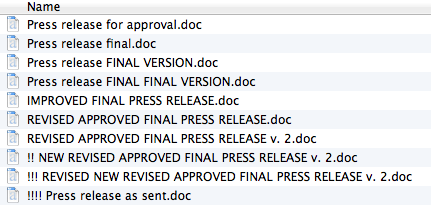
1.3 The CompSci Group Project issue
- Your group project is due tonight and your code is ready for submission
- While running a final test (for good luck) you discover a minor bug your group member forgot about
- You accidentally changed working code and ended up breaking a chunk of code you don’t understand
- You no longer remember what was and wasn’t there
- It is 23:58
1.4 An actual physics report I was working on
- I’m a last minute person, report is due tomorrow
- It’s manageable, I typed up \(\frac{2}{3}\) of it yesterday
- I’m moving an image in the Discussion section around, Microsoft Word freezes
- I wait a few minutes, oh well, looks like I need to Force Quit
- Re-open the document: Word complains that it’s corrupted, and that it can’t restore the file
- I look at my
Report v3.docx
, it’s from several hours ago - I need to re-do at least half of the work I’ve done
- I settle in for a later night than I was planning on…
2 Introduction
2.1 Version control systems — recording changes to files over time
It keeps track of changes.
It keeps track of changes really well.
- My changes
- Your group member’s changes
- The version that’s been sent to the customer
- The version that’s being tested with an urgent bugfix
- The new experimental version that might break everything else
- Whatever other changes have happened…
2.2 What is Git?
- Open source project circa. 2005 (developed for the Linux kernel)
- A command line utility (but there are graphical interfaces)
- Imagine
git
as something sitting on top of your file system - A distributed version control system —
DVCS
- Keeps all change info in a
.git
folder
2.3 Aside: Distributed Version Control Systems (DVCS)
A thing that solves our earlier problems
- Version control system — A system recording changes to files over time
- Distributed — No main server, full history available to anyone
2.4 Git: A structured way of storing versions and changes
- Every change is stored as a new compressed file, or a difference to a previous version
- This creates a structure known as a DAG
git operates on —and provides an interface to— this structure
2.5 What does this mean?
You’ll have no reason to ever lose your work again, and unparalleled ability to traverse and review changes made.
3 Getting started
3.1 Setup
- Download git
- There are nice graphical interfaced, but we’ll introduce those later
- The git commands are universal, and the simplest way to get started
3.2 Creating a repository (i.e. project)
Create a new directory (e.g. my big project
), open a terminal within it and
execute
git init
Congratulations, you’ve created a repository
3.3 Clone a repository
Want to build on somebody else’s work? That’s easy!
git clone /path/to/repository
This also works with remote servers, e.g. git clone
https://github.com/tecosaur/vscode-settings.git
.
3.4 Workflow
Local repo contains three trees in the .git file
- Working directory - Where all the files are
- Index - The staging area
- HEAD - The latest commit
3.5 Add and commit
After changing some files you add it to the index tree
git add <filename>
git add *
Then to actually commit them to the HEAD
git commit -m "Message"
All commits need a message and are given an identifying serial number, which is a hash of its structure and contents.
Your changes are still local however
3.6 What’s with these messages?
There is no hard and fast rule but the idea is to describe what this commit does
- Adds support for x. Fixes bug that caused y.
- Removes changes made in commit z.
- Add discussion of errors to report
Looking at a list of commit messages should give a decent idea of what happened to the code. Try to improve on the following commit messages:
- General commit
- Made some changes
- Bug-fixes
- Debugging 4: Reloaded (continued)
- I’ve actually done this before
3.7 So, what are we doing?
Git tracks the changes between files
- Additions
- Deletions
Starting from a HEAD and given a sequence of commits, you could follow what each says and end up at the same final point
4 The next few steps
4.1 Pushing and Pulling
To send your committed changes back to someone else’s copy
git push origin <branch>
And to fetch some new changes
git pull
4.2 Branching
Used to develop features isolated from the main code (remember your tragic mistake just before submitting?) master is the default branch. To create a new branch named “feature”
git checkout -b feature
Switch back to master
git checkout master
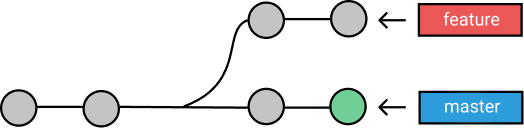
4.3 Branching
A branch — or any other change — is not available to others unless you push it to your remote repository
git push origin <branch>
4.4 Merging
To bring down any changes made by someone or somewhere else
git pull
To merge another branch into your current branch (e.g. master)
git merge <branch>
This will attempt to merge any changes from <branch> into the branch your current HEAD is on

4.5 Merge Conflicts
In both cases git
tries to auto-merge changes. Conflicts — two different
changes to the same part of the same file — need to be resolved
manually and added before trying again.
git add <filename>
One can also preview changes (to see if conflicts will occur)
git diff <source_branch> <target_branch>
\hfill

4.6 Tagging
When you hit a major milestone (it works, we’ve submitted etc.) you can tag a particular commit
git tag 1.0.0 2b331ie545
The 2b…
is the first 10 characters of the commit id you want to reference.
You can find a commit id by looking at the log.
4.7 Log
You can study a repository history using git log There are many nice options
git log --pretty=oneline
Or maybe you’d like to see some ASCII art
git log --graph --oneline --decorate --all
See only which files have changed
git log --name-status
git log --help
4.8 Oooops
Help, I destroyed EVERYTHING
All the files are gone, I’ve replaced them with .jpeg
s of Nicholas Cage, I’m
tired and in need of a hug
I wish I could go back in time
4.9 No problem!
I wish I could go back in time
You can! You can replace local changes with
git checkout -- <filename>
Or if you’ve committed a bunch of stuff that’s wrong and just want to copy from
the main repository — you could git clone
a new copy, or you can
git fetch origin
git reset --hard origin/master
5 Summary
5.1 Summary
- Git tracks changes to files
- Changes are stored as commits (cheap and plentiful)
- A group of commits can be pushed (setting them in stone — kinda)
- Branches allow you to develop new things in isolation
- Can be merged back together again when finished
- You can move through time and code together
There’s a lot more you can recover from